React Data Flow
React's philosophy is simple: the user (with a click, etc.) triggers the actions that modify the state of your App. This modification, in turn, alters the UI.
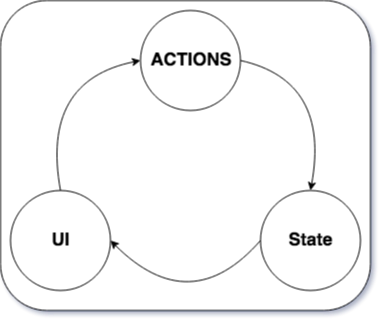
This pattern also fits well with React's unidirectional data flow. The general idea is that data goes down (from parent to child components) while actions (updates) go up, effectively creating a circular system.
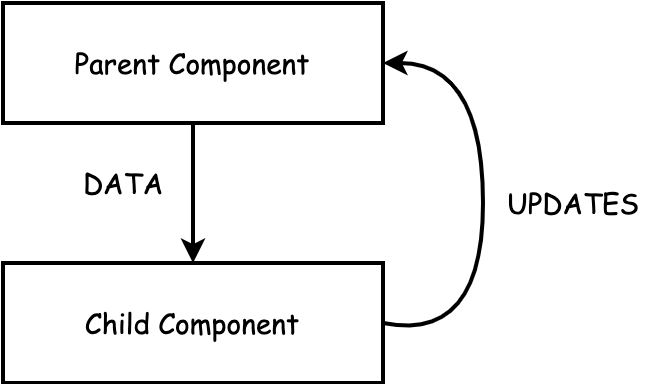
In React, data only flows in one direction: from parent to child. So when data gets updated in the parent, React will pass it to all the children (as props) who use it.
Often, several components need to reflect the same changing data. Therefore, it is recommended to lift the shared state up to their closest common ancestor.
In such cases, you should not duplicate the state in the child component. If you find yourself passing down props from the parent to generate state in the child component, pause and rethink. It is generally considered an anti-pattern to have a situation exhibited in the code below.
class Savings extends React.Component {
constructor(props) {
super(props);
this.state = {
balance: this.props.balance
};
}
render() {
return (
<div>
Your balance is {this.state.balance}
</div>
);
}
}